In the realm of modern software development, microservices architecture has garnered immense popularity for its ability to construct scalable and resilient applications. When integrated with reactive programming principles, microservices gain even greater potency, adeptly managing high-throughput and low-latency scenarios. This guide delves into harnessing Spring Boot and Java for crafting reactive microservices, offering a comprehensive overview and practical insights into building robust reactive systems.
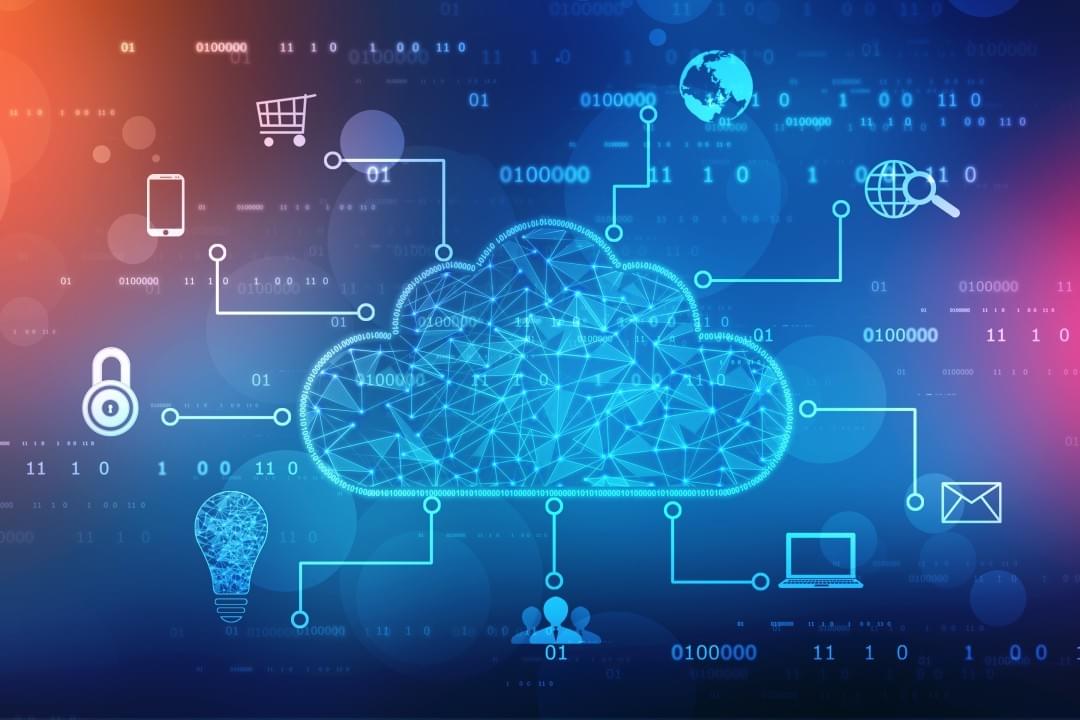
What are Microservices?
Microservices architecture divides large, monolithic applications into smaller, independently deployable services. Each service focuses on a specific business capability and communicates with others through lightweight mechanisms like HTTP/REST or messaging protocols such as Kafka or RabbitMQ.
Why Reactive?
Reactive programming is a paradigm emphasising asynchronous and non-blocking operations, designed to efficiently handle numerous concurrent users and events. Key attributes of reactive systems include responsiveness, resilience, elasticity, and message-driven communication.
Benefits of Reactive Microservices
- Scalability: Easily scale individual microservices based on demand.
- Resilience: Gracefully handle failures through built-in fault tolerance mechanisms.
- Responsiveness: React promptly to user requests and events.
- Efficiency: Optimise resource utilisation with non-blocking I/O operations.
Getting Started with Spring Boot and Java
Setting Up Your Development Environment
Before delving into coding, ensure the following tools are installed:
- Java Development Kit (JDK): Download and install the latest JDK from Oracle or OpenJDK.
- Integrated Development Environment (IDE): Utilize IntelliJ IDEA, Eclipse, or your preferred IDE for Java development.
- Spring Boot: Simplifies setup and development of Spring applications; effortlessly integrate essential dependencies using Spring Boot starters.
Project Setup
Let's initiate a basic Spring Boot project for our reactive microservice:
- Initialise a Spring Boot Project: Use Spring Initializr or your IDE to create a unique Spring Boot project with dependencies like WebFlux and Spring Data MongoDB or Reactive SQL.
- Configure Dependencies: Add dependencies for WebFlux (reactive web framework), Spring Data Reactive MongoDB or Reactive SQL (for reactive data access), and any other need dependencies like Spring Cloud Netflix (for service discovery and configuration).
Building Reactive Microservices
Reactive Programming Basics
Reactive programming in Java typically involves:
- Flux and Mono: Core types in Project Reactor, the reactive library used by Spring WebFlux.
- Schedulers: Manage concurrency and threading in reactive applications.
Implementing Reactive Endpoints
- Controller Layer: Define RESTful endpoints using annotated controllers to handle requests using reactive types.
- Service Layer: Implement business logic using reactive programming constructs such as mapping, filtering, and flat-mapping operations on reactive streams.
Reactive Data Access
- Repository Layer: Employ Spring Data Reactive repositories to interact asynchronously with databases. For instance, utilise ReactiveMongoRepository for MongoDB or R2dbcRepository for Reactive SQL databases.
Reactive Streams and Messaging
- Event-Driven Communication: Employ messaging queues like Kafka or RabbitMQ for asynchronous communication between microservices.
- Integration with Reactive Streams: Spring Integration facilitates integrating reactive applications with diverse messaging systems.
Best Practices for Reactive Microservices
Design Principles
- Domain-Driven Design (DDD): Partition responsibilities based on business domains to create cohesive and loosely coupled microservices.
- Circuit Breaker Pattern: Implement fault tolerance and resilience using patterns such as Hystrix or Resilience4j.
Operational Considerations
- Monitoring and Observability: Utilize tools like Prometheus, Grafana, or Spring Boot Actuator to monitor reactive microservices.
- Deployment Strategies: Deploy microservices using containerization (Docker) and orchestration (Kubernetes) for scalability and resilience.
Testing
- Unit Testing: Test reactive components with tools like JUnit and Mockito to mock reactive dependencies.
- Integration Testing: Validate interaction between microservices and external dependencies with tools like Spring Boot Test and Testcontainers.
Conclusion
Reactive microservices with Spring Boot and Java are key for building scalable and resilient applications in today's tech landscape. By leveraging reactive programming principles, developers can achieve high performance and efficiency in handling concurrent requests. Whether starting a new project or transitioning to microservices, mastering reactive programming with Spring Boot and Java prepares you to tackle modern application challenges effectively. Enhance your skills with Java certification course in Greater Noida, Delhi, Pune, and other Indian cities. These courses offer structured learning, cover advanced topics, provide expert guidance, and include practical exercises in Java programming and reactive microservices. Keep exploring and applying these concepts to stay ahead in software engineering.