Functional programming is a paradigm that emphasises writing code using functions that are predictable and do not alter external states. Its focus on immutability and pure functions has made it highly relevant in modern software development, leading to more robust and maintainable code. Although Java has traditionally been known for its object-oriented approach, it has integrated functional programming concepts, particularly from Java 8 onward. This article explores how Java incorporates functional programming, its core principles, and its practical advantages.
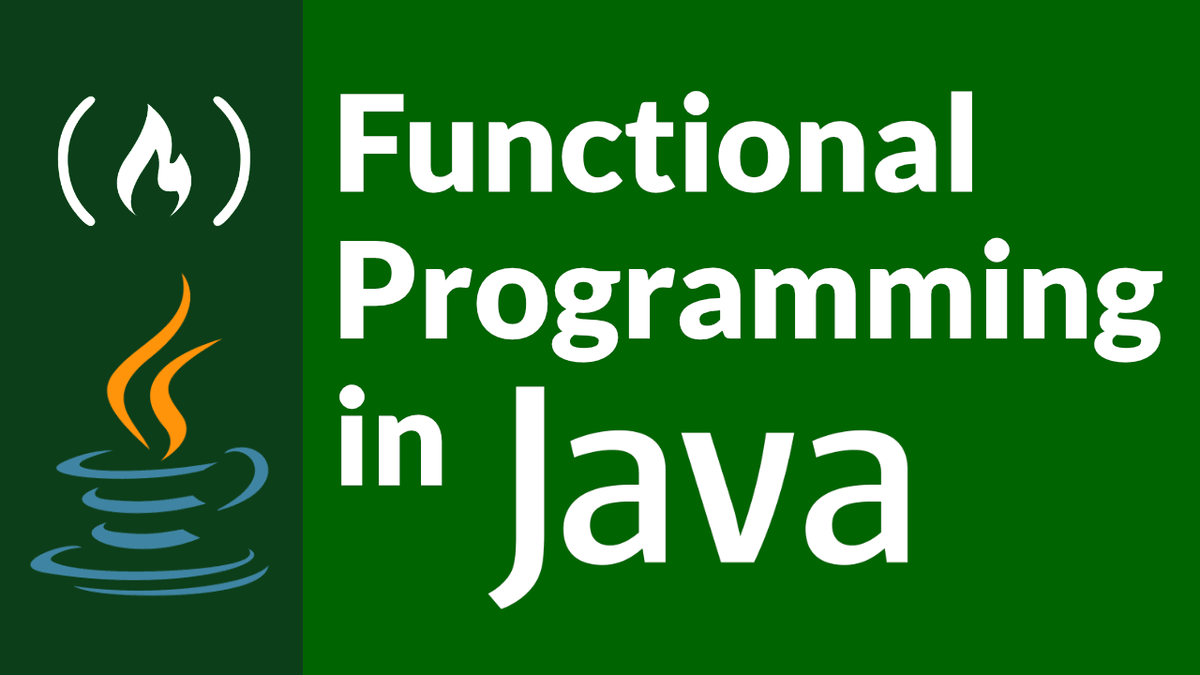
1. Pure Functions
In functional programming, a pure function is one that consistently produces the same output for the same input and has no side effects. This means that a pure function does not modify any external state or depend on any mutable state. Because of their predictable behavior, pure functions are easier to reason about, test, and debug.
2. Immutability
Immutability refers to objects whose state cannot be changed once they are created. Functional programming often uses immutable data structures to prevent unintended side effects from data modifications. This approach also simplifies concurrent programming by eliminating issues related to mutable shared states, making applications more reliable and easier to manage.
3. First-Class Functions
In functional programming, functions are treated as first-class citizens. This means that functions can be passed as arguments, returned as results, and assigned to variables. This flexibility allows for more abstract and reusable code, promoting a more modular and adaptable approach to programming.
4. Higher-Order Functions
Higher-order functions are those that can accept other functions as arguments or return them as results. This capability supports code abstraction and reuse, enabling developers to write more modular and composable code. Higher-order functions help in creating more flexible and generic solutions to various programming problems.
Functional Programming Features in Java
Java has embraced functional programming concepts, particularly with the release of Java 8. Key features include:
1. Lambda Expressions
Lambda expressions offer a concise way to represent anonymous functions or behaviors. They simplify the implementation of interfaces with a single abstract method, reducing boilerplate code and enhancing readability. Lambda expressions make it easier to pass around and execute code blocks in a streamlined manner.
2. Functional Interfaces
A functional interface in Java is an interface with exactly one abstract method. Although these interfaces can have multiple default or static methods, they are defined by their single abstract method. Java’s standard library includes several built-in functional interfaces, such as Function, Predicate, Consumer, and Supplier. These interfaces facilitate functional programming by enabling the use of lambda expressions and method references.
3. Streams API
The Streams API enables functional-style operations on sequences of elements, such as collections. Streams support operations like filtering, mapping, and reducing in a fluent and declarative manner. This API helps process data more efficiently and readably by leveraging lazy evaluation and parallel processing.
4. Optional Class
The Optional class is a container object that may or may not contain a value. It provides a way to handle cases where a value might be absent without relying on null references. Optional helps avoid NullPointerException and promotes more explicit handling of optional values.
Benefits of Functional Programming in Java
1. Improved Readability and Maintainability
Functional programming promotes writing clear and concise code. By focusing on pure functions and immutable data, code becomes easier to understand and maintain. The declarative nature of functional programming makes the intention of the code more apparent.
2. Enhanced Testing and Debugging
Pure functions and immutable data structures simplify testing and debugging. Since pure functions consistently produce the same result for the same input and do not have side effects, they can be tested in isolation. This predictability makes it easier to identify and fix issues, leading to more reliable software.
3. Simplified Concurrency and Parallelism
Functional programming principles, such as immutability and stateless functions, make concurrent and parallel programming more manageable. With immutable data structures, there are fewer concerns about race conditions and data consistency, resulting in more robust and scalable applications.
4. Greater Reusability and Composability
Higher-order functions and functional interfaces enhance code reusability and composability. Functions can be easily passed around, combined, and reused in different contexts, leading to more flexible and adaptable code structures.
Practical Applications
1. Data Processing
The Streams API is particularly useful for data processing tasks, such as transforming, filtering, and aggregating collections of data. It allows developers to write expressive and efficient code, making data manipulation more streamlined and manageable.
2. Event Handling
Lambda expressions and functional interfaces simplify event handling in Java. For instance, in GUI applications or event-driven systems, lambda expressions can be used to define event listeners and handlers more succinctly, reducing code verbosity and improving clarity.
3. Functional Programming in APIs
Modern Java libraries and frameworks increasingly adopt functional programming principles. For example, Java’s standard library and frameworks like Spring encourage the use of functional interfaces and streams, leading to cleaner and more efficient code.
Conclusion
Java’s adoption of functional programming features has modernised the language and broadened its capabilities. By incorporating concepts like lambda expressions, functional interfaces, and the Streams API, Java developers can write more expressive, maintainable, and efficient code. Understanding and applying functional programming principles can enhance Java applications and improve overall programming practices. As software development evolves, leveraging functional programming techniques in Java will continue to be a valuable skill. For comprehensive learning and skill development, seeking out a reputable Java course provider in Gurgaon, Mumbai, Pune and other cities across India can offer additional insights and practical experience in functional programming.