Functional Programming in Java: A Modern Approach
Functional Programming in Java: A Modern Approach
Transforming Java Development with Functional Techniques for Enhanced Readability and Efficiency
Introduction
Functional programming (FP) is a paradigm that emphasizes the evaluation of expressions rather than the execution of statements. It focuses on functions as first-class citizens, treating them as values that can be passed around and manipulated. This approach often leads to more concise, readable, and maintainable code.
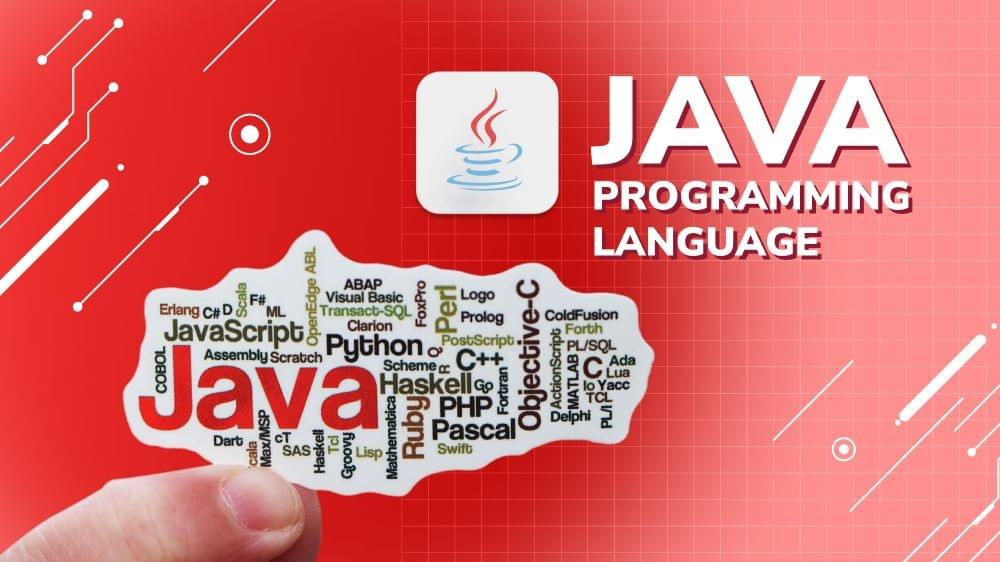
Key Concepts of Functional Programming
- Immutability: Values should be immutable, meaning they cannot be changed after creation. This helps prevent side effects and makes code easier to reason about.
- Pure Functions: Functions should have no side effects and always return the same output for a given input. This makes them easier to test and reuse.
- Higher-Order Functions: Functions can be passed as arguments to other functions or returned as results. This enables powerful abstractions and code reuse.
- Lazy Evaluation: Expressions are evaluated only when their results are needed. This can improve performance in certain scenarios.
Functional Features in Java
Java 8 introduced several features that support functional programming:
- Lambda Expressions: These are anonymous functions that can be used to represent expressions.
- Method References: They provide a concise way to refer to methods or constructors.
- Streams: A powerful API for processing collections of elements in a declarative style.
- Optional: A container for a single optional value. It helps handle null values in a more robust way.
Example: Using Lambda Expressions
Java
List names = Arrays.asList("Alice", "Bob", "Charlie");
// Traditional approach
List uppercaseNames = new ArrayList<>();
for (String name : names) {
uppercaseNames.add(name.toUpperCase());
}
// Functional approach using lambda expressions
List uppercaseNames2 = names.stream()
In the functional approach, we use a stream to process the list of names. The map operation applies the toUpperCase function to each element, and the collect operation collects the results into a new list.
The stream API provides various operations for filtering, mapping, reducing, and collecting elements. In this example, we use max to find the maximum element based on the age comparator.
Conclusion
Functional programming can be a powerful tool for writing concise, readable, and maintainable Java code. By leveraging the features introduced in Java 8, you can incorporate functional techniques into your projects and benefit from their advantages. Exploring these concepts is particularly useful for individuals engaging in java training classes in Delhi, Noida, Pune and other Indian cities as it offers a different perspective on problem-solving. While functional programming may not be suitable for all use cases, it's worth considering for enhancing your coding skills.